If you ever needed a comprehensive reference on Warband Module System operations, you have found it!
Operations introduced in Warband patches 1.153-1.166 are indicated appropriately.
Here you can find a thoroughly restructured, expanded and documented version of header_operations.py file from the Warband Module System. All operations have been grouped into sections, their calling syntax has been checked, nearly every operation has been properly commented. Many mistakes or inconsistencies in operation calling syntax or supposed effects have been researched and corrected. Most unobvious conventions and assumptions are highlighted.
What's more, a lot of comments have been added to the file which should make it much easier to use by people who are still learning the ropes of Warband modding.
Download URL #1: http://www.nexusmods.com/mountandblade/mods/3478
Download URL #2: http://www.mbrepository.com/file.php?id=3478
Updated version by Earendil at GitLab:
gitlab.com
Operators Documentation by Vetrogor at mbcommands.fandom:
mbcommands.fandom.com
The file still could be improved in some places, and not all operations have been completely researched to this moment. Multiplayer section in particular has not been expanded at all, as I do not consider myself an expert on Warband MP modding. Tableau section could use some extra love as well. Some inaccuracies in other sections are also possible, humans do make mistakes after all. Despite all that, I daresay this is the file which will be of great help to any Warband scripter.
Special credits go to cmpxchg8b, Caba'drin, SonKidd, MadVader, dunde, Ikaguia, MadocComadrin, Cjkjvfnby and shokkueibu for their help and support during this file development.
Operations introduced in Warband patches 1.153-1.166 are indicated appropriately.
Here you can find a thoroughly restructured, expanded and documented version of header_operations.py file from the Warband Module System. All operations have been grouped into sections, their calling syntax has been checked, nearly every operation has been properly commented. Many mistakes or inconsistencies in operation calling syntax or supposed effects have been researched and corrected. Most unobvious conventions and assumptions are highlighted.
What's more, a lot of comments have been added to the file which should make it much easier to use by people who are still learning the ropes of Warband modding.
Download URL #1: http://www.nexusmods.com/mountandblade/mods/3478
Download URL #2: http://www.mbrepository.com/file.php?id=3478
Updated version by Earendil at GitLab:
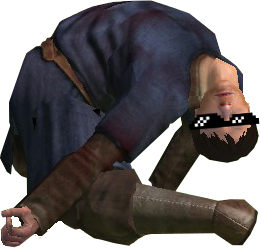
Limik / header_operations · GitLab
Documentation and Descriptions of the M&B Engine Operations.

Operators Documentation by Vetrogor at mbcommands.fandom:
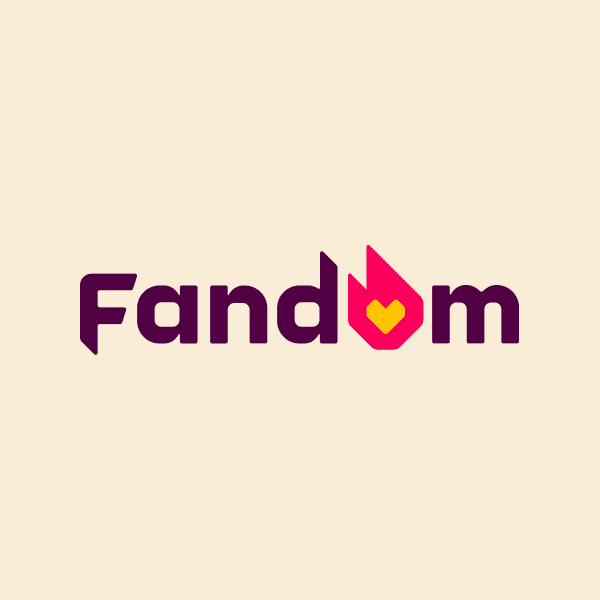
Operators
This reference created from updated LAV's header_operations.py. Changes are made by me, Vetrogor. If you want to add or edit information PM me on the forum or discord. Special thanks for Dalion who is also the main contributer. Information from Earandil git header_operations.py is added. He...
Code:
################################################################################
# header_operations expanded RC1 #
################################################################################
# TABLE OF CONTENTS
################################################################################
#
# [ Z00 ] Introduction and Credits.
# [ Z01 ] Operation Modifiers.
# [ Z02 ] Flow Control.
# [ Z03 ] Mathematical Operations.
# [ Z04 ] Script/Trigger Parameters and Results.
# [ Z05 ] Keyboard and Mouse Input.
# [ Z06 ] World Map.
# [ Z07 ] Game Settings.
# [ Z08 ] Factions.
# [ Z09 ] Parties and Party Templates.
# [ Z10 ] Troops.
# [ Z11 ] Quests.
# [ Z12 ] Items.
# [ Z13 ] Sounds and Music Tracks.
# [ Z14 ] Positions.
# [ Z15 ] Game Notes.
# [ Z16 ] Tableaus and Heraldics.
# [ Z17 ] String Operations.
# [ Z18 ] Output And Messages.
# [ Z19 ] Game Control: Screens, Menus, Dialogs and Encounters.
# [ Z20 ] Scenes and Missions.
# [ Z21 ] Scene Props and Prop Instances.
# [ Z22 ] Teams and Agents.
# [ Z23 ] Presentations.
# [ Z24 ] Multiplayer And Networking.
# [ Z25 ] Remaining Esoteric Stuff.
# [ Z26 ] Hardcoded Compiler-Related Code.
#
################################################################################
Code:
################################################################################
# [ Z14 ] POSITIONS
################################################################################
# Positions are the 3D math of the game. If you want to handle objects in
# space, you will inevitably have to deal with positions. Note that while most
# position-handling operations work both on global map and on the scenes,
# there are operations which will only work in one or another mode.
# Each position consists of three parts: coordinates, rotation and scale.
# Coordinates are three numbers - (X,Y,Z) - which define a certain point in
# space relative to the base of coordinates. Most of the time, the base of
# coordinates is either the center of the global map, or the center of the
# scene, but there may be exceptions. Note that all operations with
# coordinates nearly always use fixed point numbers.
# Position rotation determines just that - rotation around corresponding
# world axis. So rotation around Z axis means rotation around vertical axis,
# in other words - turning right and left. Rotation around X and Y axis will
# tilt the position forward/backwards and right/left respectively.
# It is common game convention that X world axis points to the East, Y world
# axis points to the North and Z world axis points straight up. However this
# is so-called global coordinates system, and more often than not you'll be
# dealing with local coordinates. Local coordinates are the coordinate system
# defined by the object's current position. For the object, his X axis is to
# the right, Y axis is forward, and Z axis is up. This is simple enough, but
# consider what happens if that object is turned upside down in world space?
# Object's Z axis will point upwards *from the object's point of view*, in
# other words, in global space it will be pointing *downwards*. And if the
# object is moving, then it's local coordinates system is moving with it...
# you get the idea.
# Imagine the position as a small point with an arrow somewhere in space.
# Position's coordinates are the point's position. Arrow points horizontally
# to the North by default, and position's rotation determines how much was
# it turned in the each of three directions.
# Final element of position is scale. It is of no direct relevance to the
# position itself, and it does not participate in any calculations. However
# it is important when you retrieve or set positions of objects. In this
# case, position's scale is object's scale - so you can shrink that wall
# or quite the opposite, make it grow to the sky, depending on your whim.
# Generic position operations
init_position = 701 # (init_position, <position>),
# Sets position coordinates to [0,0,0], without any rotation and default scale.
copy_position = 700 # (copy_position, <position_target>, <position_source>),
# Makes a duplicate of position_source.
position_copy_origin = 719 # (position_copy_origin, <position_target>, <position_source>),
# Copies coordinates from source position to target position, without changing rotation or scale.
position_copy_rotation = 718 # (position_copy_rotation, <position_target>, <position_source>),
# Copies rotation from source position to target position, without changing rotation or scale.
position_transform_position_to_parent = 716 # (position_transform_position_to_parent, <position_dest>, <position_anchor>, <position_relative_to_anchor>),
# Converts position from local coordinate space to parent coordinate space. In other words, if you have some position on the scene (anchor) and a position describing some place *relative* to anchor (for example [10,20,0] means "20 meters forward and 10 meters to the right"), after calling this operation you will get that position coordinates on the scene in <position_dest>. Rotation and scale is also taken care of, so you can use relative angles.
position_transform_position_to_local = 717 # (position_transform_position_to_local, <position_dest>, <position_anchor>, <position_source>),
# The opposite to (position_transform_position_to_parent), this operation allows you to get source's *relative* position to your anchor. Suppose you want to run some decision making for your bot agent depending on player's position. In order to know where player is located relative to your bot you call (position_transform_position_to_local, <position_dest>, <bot_position>, <player_position>). Then we check position_dest's Y coordinate - if it's negative, then the player is behind our bot's back.
# Position (X,Y,Z) coordinates
position_get_x = 726 # (position_get_x, <destination_fixed_point>, <position>),
# Return position X coordinate (to the east, or to the right). Base unit is meters. Use (set_fixed_point_multiplier) to set another measurement unit (100 will get you centimeters, 1000 will get you millimeters, etc).
position_get_y = 727 # (position_get_y, <destination_fixed_point>, <position>),
# Return position Y coordinate (to the north, or forward). Base unit is meters. Use (set_fixed_point_multiplier) to set another measurement unit (100 will get you centimeters, 1000 will get you millimeters, etc).
position_get_z = 728 # (position_get_z, <destination_fixed_point>, <position>),
# Return position Z coordinate (to the top). Base unit is meters. Use (set_fixed_point_multiplier) to set another measurement unit (100 will get you centimeters, 1000 will get you millimeters, etc).
position_set_x = 729 # (position_set_x, <position>, <value_fixed_point>),
# Set position X coordinate.
position_set_y = 730 # (position_set_y, <position>, <value_fixed_point>),
# Set position Y coordinate.
position_set_z = 731 # (position_set_z, <position>, <value_fixed_point>),
# Set position Z coordinate.
position_move_x = 720 # (position_move_x, <position>, <movement>, [value]),
# Moves position along X axis. Movement distance is in cms. Optional parameter determines whether the position is moved along the local (value=0) or global (value=1) X axis (i.e. whether the position will be moved to it's right/left, or to the global east/west).
position_move_y = 721 # (position_move_y, <position>, <movement>,[value]),
# Moves position along Y axis. Movement distance is in cms. Optional parameter determines whether the position is moved along the local (value=0) or global (value=1) Y axis (i.e. whether the position will be moved forward/backwards, or to the global north/south).
position_move_z = 722 # (position_move_z, <position>, <movement>,[value]),
# Moves position along X axis. Movement distance is in cms. Optional parameter determines whether the position is moved along the local (value=0) or global (value=1) Z axis (i.e. whether the position will be moved to it's above/below, or to the global above/below - these directions will be different if the position is tilted).
position_set_z_to_ground_level = 791 # (position_set_z_to_ground_level, <position>),
# This will bring the position Z coordinate so it rests on the ground level (i.e. an agent could stand on that position). This takes scene props with their collision meshes into account. Only works during a mission, so you can't measure global map height using this.
position_get_distance_to_terrain = 792 # (position_get_distance_to_terrain, <destination>, <position>),
# This will measure the distance between position and terrain below, ignoring all scene props and their collision meshes. Operation only works on the scenes and cannot be used on the global map.
position_get_distance_to_ground_level = 793 # (position_get_distance_to_ground_level, <position>),
# This will measure the distance between position and the ground level, taking scene props and their collision meshes into account. Operation only works on the scenes and cannot be used on the global map.
Terms of Use said:You are free to use this file in your module sources.
You are also free to redistribute the file (separately or as part of your module sources), use the information from the file to create other forms of Module System scripting reference, or convert it to other formats (CHM, HTML, DOC, RTF, PDF, whatever) for as long as you keep "Introduction and Credits" section intact. You can add to it, highlighting your own contribution to the project, but never remove.
The file still could be improved in some places, and not all operations have been completely researched to this moment. Multiplayer section in particular has not been expanded at all, as I do not consider myself an expert on Warband MP modding. Tableau section could use some extra love as well. Some inaccuracies in other sections are also possible, humans do make mistakes after all. Despite all that, I daresay this is the file which will be of great help to any Warband scripter.
Special credits go to cmpxchg8b, Caba'drin, SonKidd, MadVader, dunde, Ikaguia, MadocComadrin, Cjkjvfnby and shokkueibu for their help and support during this file development.
Last edited by a moderator: