kefka95
Squire
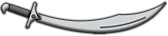
Does anyone know how to convert from the compiled item_kinds decimal representation of flags to the individual flags themselves? I know how to come up with the decimal number given the flags (ie, by adding the hex values of the constants), but I don't know how to do it the other way around.
For example, I know that 4194309 represents itp_type_arrows + itp_primary. If I were given the 4194309 without any foreknowledge of what flags that represented, how would I figure out that that number breaks down to itp_type_arrows + itp_primary? The game can obviously do it, and other editors can as well, but I'm too slow to figure it out myself. I'm sure there's some "mathy" way of doing it, but that's not exactly my strong suit
.
Any help would be appreciated!
For example, I know that 4194309 represents itp_type_arrows + itp_primary. If I were given the 4194309 without any foreknowledge of what flags that represented, how would I figure out that that number breaks down to itp_type_arrows + itp_primary? The game can obviously do it, and other editors can as well, but I'm too slow to figure it out myself. I'm sure there's some "mathy" way of doing it, but that's not exactly my strong suit
Any help would be appreciated!