Karsontr
Regular
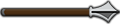
I had Chat AI write a code for the warband so that the lords would age and die, and be replaced by a son from a family member after he dies. Do you think this code module can be integrated into the system and will it work in the game?
Chat Aİ:
In order to write the necessary codes for the aging and death of lords, we must add a class to the module system that will store the age of the lords and update it when any event occurs. The structure of this class could be:
Features of this class include keeping lords' names and ages. The increase_age() method is used to update the ages of the lords.
Now we can write the codes for the death of the old lords and the replacement of their sons. For this, you can add a death_chance() method to the Lord class and have this method called as it ages. As a result of this method working, lords who have reached a certain age can be made to die with a random probability. We can also add a special code for the replacement of deceased lords by their sons. In this code, the deceased lord can be replaced by his son of the same name.
In these code examples, we added a sons property to the Lord class. This attribute is used to hold sons of lords. If there is no lord son, nothing is done because no one can replace him.
For example, at the end of each term, as we increase the age of each lord, we can see that the dead lords are replaced by their sons.
Chat Aİ:
In order to write the necessary codes for the aging and death of lords, we must add a class to the module system that will store the age of the lords and update it when any event occurs. The structure of this class could be:
```
class Lord:
def __init__(self, name, age):
self.name = name
self.age = age
def get_age(self):
return self.age
def set_age(self, age):
self.age = age
def increase_age(self):
self.age += 1
```
Features of this class include keeping lords' names and ages. The increase_age() method is used to update the ages of the lords.
Now we can write the codes for the death of the old lords and the replacement of their sons. For this, you can add a death_chance() method to the Lord class and have this method called as it ages. As a result of this method working, lords who have reached a certain age can be made to die with a random probability. We can also add a special code for the replacement of deceased lords by their sons. In this code, the deceased lord can be replaced by his son of the same name.
```
import random
class Lord:
def __init__(self, name, age):
self.name = name
self.age = age
self.sons = []
def get_age(self):
return self.age
def set_age(self, age):
self.age = age
def increase_age(self):
self.age += 1
self.death_chance()
def death_chance(self):
if self.age >= 60: #10% chance of death for 60 year old lords
chance = random.randint(1, 10)
if chance == 1:
print(f'{self.name} has died of natural causes.')
self.replace_with_son()
def replace_with_son(self):
if self.sons:
# random.randint() makes a random choice between sons.
#so that a certain son of the lord will pass.
son = random.randint(0, len(self.sons)-1)
new_lord = self.sons[son]
print(f'{new_lord.name} has taken over the throne!')
```
In these code examples, we added a sons property to the Lord class. This attribute is used to hold sons of lords. If there is no lord son, nothing is done because no one can replace him.
For example, at the end of each term, as we increase the age of each lord, we can see that the dead lords are replaced by their sons.